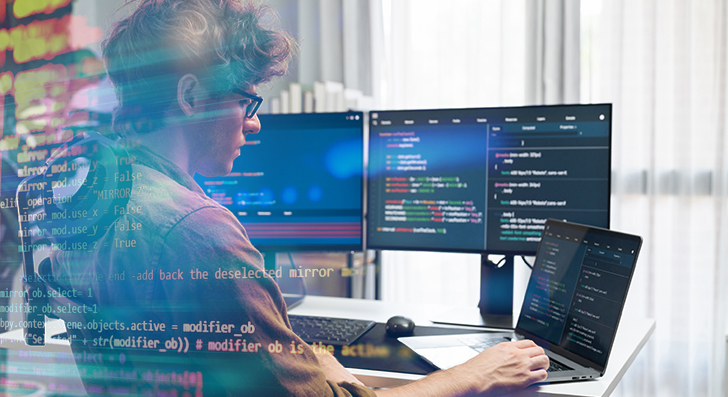
Scalability suggests your software can cope with expansion—a lot more customers, extra facts, plus more website traffic—devoid of breaking. As being a developer, building with scalability in your mind saves time and strain later on. Here’s a transparent and sensible guideline to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it ought to be portion of your plan from the start. Many apps fail whenever they develop rapid since the first style can’t handle the extra load. As a developer, you must think early about how your procedure will behave under pressure.
Get started by developing your architecture for being adaptable. Avoid monolithic codebases the place all the things is tightly linked. In its place, use modular layout or microservices. These styles break your app into lesser, independent areas. Each module or services can scale By itself without the need of affecting The full process.
Also, consider your databases from working day 1. Will it want to manage one million customers or maybe 100? Select the right sort—relational or NoSQL—based upon how your details will grow. Program for sharding, indexing, and backups early, Even though you don’t need to have them nonetheless.
An additional essential place is to stay away from hardcoding assumptions. Don’t write code that only functions below existing situations. Contemplate what would materialize In the event your person base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that aid scaling, like information queues or celebration-pushed programs. These support your application handle more requests without getting overloaded.
When you build with scalability in your mind, you are not just making ready for fulfillment—you are lowering potential head aches. A effectively-planned method is easier to maintain, adapt, and mature. It’s superior to arrange early than to rebuild afterwards.
Use the appropriate Database
Choosing the ideal databases can be a important part of setting up scalable apps. Not all databases are designed the exact same, and using the wrong you can slow you down or simply bring about failures as your app grows.
Get started by comprehension your info. Is it really structured, like rows in the table? If Certainly, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and regularity. They also assist scaling methods like browse replicas, indexing, and partitioning to deal with more targeted traffic and data.
If the information is much more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and can scale horizontally far more very easily.
Also, take into consideration your study and produce patterns. Do you think you're accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a heavy compose load? Take a look at databases that may take care of higher produce throughput, or perhaps party-primarily based data storage programs like Apache Kafka (for short term facts streams).
It’s also smart to Feel forward. You might not have to have advanced scaling capabilities now, but deciding on a databases that supports them means you won’t will need to modify afterwards.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your data dependant upon your access patterns. And usually keep an eye on databases performance as you grow.
In short, the proper database depends upon your app’s structure, velocity requires, And exactly how you anticipate it to develop. Consider time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, each individual small hold off provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your method. That’s why it’s important to Establish successful logic from the start.
Begin by composing clean, simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward just one operates. Keep your capabilities limited, targeted, and straightforward to check. Use profiling tools to uncover bottlenecks—areas where your code can take also long to operate or utilizes an excessive amount of memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Just about every query only asks for the information you truly will need. Steer clear of Find *, which fetches almost everything, and rather decide on specific fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout huge tables.
In the event you see precisely the same data currently being asked for again and again, use caching. Retailer the effects temporarily making use of applications like Redis or Memcached so you don’t really need to repeat highly-priced operations.
Also, batch your databases operations any time you can. Rather than updating a row one after the other, update them in teams. This cuts down on overhead and helps make your read more application extra effective.
Make sure to exam with large datasets. Code and queries that do the job fine with one hundred data might crash once they have to manage one million.
In short, scalable apps are quick apps. Keep your code restricted, your queries lean, and use caching when necessary. These measures support your software continue to be sleek and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and a lot more targeted traffic. If anything goes via 1 server, it's going to swiftly become a bottleneck. That’s in which load balancing and caching are available in. These two resources assist keep your application speedy, stable, and scalable.
Load balancing spreads incoming targeted visitors across numerous servers. Rather than 1 server performing all the work, the load balancer routes buyers to unique servers based upon availability. This implies no solitary server will get overloaded. If one particular server goes down, the load balancer can send out traffic to the others. Tools like Nginx, HAProxy, or cloud-centered methods from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing knowledge temporarily so it might be reused immediately. When buyers ask for the identical information all over again—like an item site or even a profile—you don’t need to fetch it within the database every time. You could provide it through the cache.
There are two prevalent forms of caching:
1. Server-aspect caching (like Redis or Memcached) shops details in memory for speedy entry.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static documents close to the person.
Caching minimizes databases load, improves velocity, and tends to make your application much more successful.
Use caching for things which don’t change generally. And usually ensure that your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but highly effective tools. Collectively, they assist your app take care of extra buyers, remain rapid, and recover from troubles. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To develop scalable purposes, you'll need equipment that permit your application grow very easily. That’s where by cloud platforms and containers can be found in. They offer you flexibility, lessen set up time, and make scaling A lot smoother.
Cloud platforms like Amazon World wide web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and products and services as you'll need them. You don’t must obtain components or guess future capacity. When traffic raises, it is possible to insert additional means with just a few clicks or automatically making use of automobile-scaling. When targeted traffic drops, you may scale down to save cash.
These platforms also present products and services like managed databases, storage, load balancing, and security resources. You'll be able to give attention to setting up your application in place of taking care of infrastructure.
Containers are A different essential Device. A container deals your app and every thing it needs to operate—code, libraries, configurations—into a person device. This can make it uncomplicated to move your app among environments, from the laptop computer for the cloud, with no surprises. Docker is the most well-liked Instrument for this.
When your application works by using a number of containers, resources like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If one particular portion of one's application crashes, it restarts it mechanically.
Containers also help it become easy to different areas of your app into products and services. It is possible to update or scale elements independently, which is perfect for overall performance and trustworthiness.
In brief, using cloud and container instruments indicates you could scale rapidly, deploy easily, and Get well quickly when challenges come about. If you would like your application to grow with no limits, start off using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on creating, not correcting.
Keep track of Almost everything
In case you don’t observe your application, you gained’t know when things go Improper. Checking helps you see how your app is doing, location problems early, and make greater conclusions as your application grows. It’s a important Component of setting up scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and companies are performing. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it's going to take for users to load pages, how frequently errors happen, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Setup alerts for essential issues. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you ought to get notified right away. This aids you repair problems quick, frequently before users even notice.
Checking is likewise handy if you make adjustments. In the event you deploy a brand new aspect and find out a spike in glitches or slowdowns, you can roll it again ahead of it triggers real destruction.
As your app grows, visitors and details raise. Without having monitoring, you’ll miss out on signs of hassle until eventually it’s also late. But with the right instruments in place, you continue to be in control.
In short, checking assists you keep the app responsible and scalable. It’s not almost spotting failures—it’s about understanding your process and ensuring it really works effectively, even stressed.
Ultimate Thoughts
Scalability isn’t only for large providers. Even tiny applications require a solid Basis. By creating thoroughly, optimizing wisely, and using the ideal equipment, you could Construct applications that grow efficiently without breaking under pressure. Start out modest, think huge, and Establish intelligent.